Enumerations are structures which resemble classes but differ from them in that in the enum body we can declare only constants. A variety of logically connected constants can be linked by means of language. These language constructs are the so-called enumerated types.
At concept level, enumerations can be declared using the C# keyword enum, instead of class:
In the above blueprint, modifiers can be public or internal, when declaring the enum directly inside a namespace, and public, internal, protected internal or private when the enum is declared inside a class. Identifier enum_name should follow rules of class naming. Finally, constants are declared inside the body of the enumeration, separated by comas.
Lets take an example of enumeration, the days of the week:
[raw][/raw]
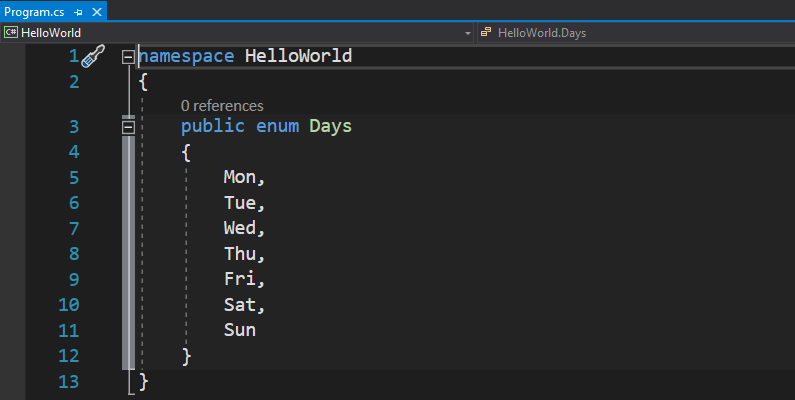
So, you can say that you have declared an enumeration of type Days, containing 7 constants named with the names of the days of the week. What you don’t know is the fact that behind the scenes, C# assigns integer values to your enumerations constants. That’s why, the above code is similar to this one:
[raw][/raw]
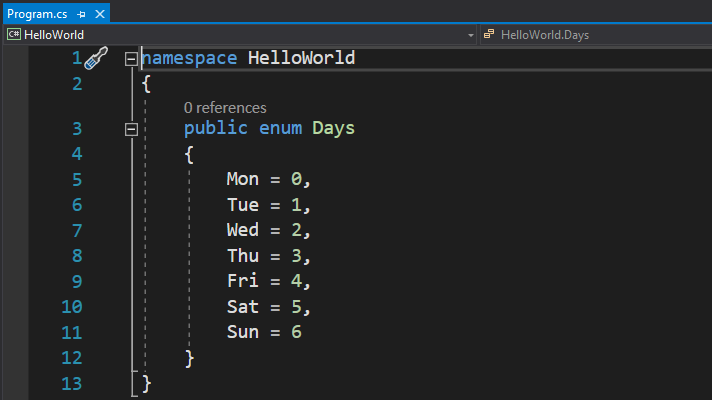
and we can test it by casting one of the enum members to an integer:
See code changes
Legend:
[/raw]
and the output would be 0. However, you cannot say that Days.Mon is of type integer, because it is not, even if it holds an integer value; it is of type Days, as we can check:
[raw][/raw]
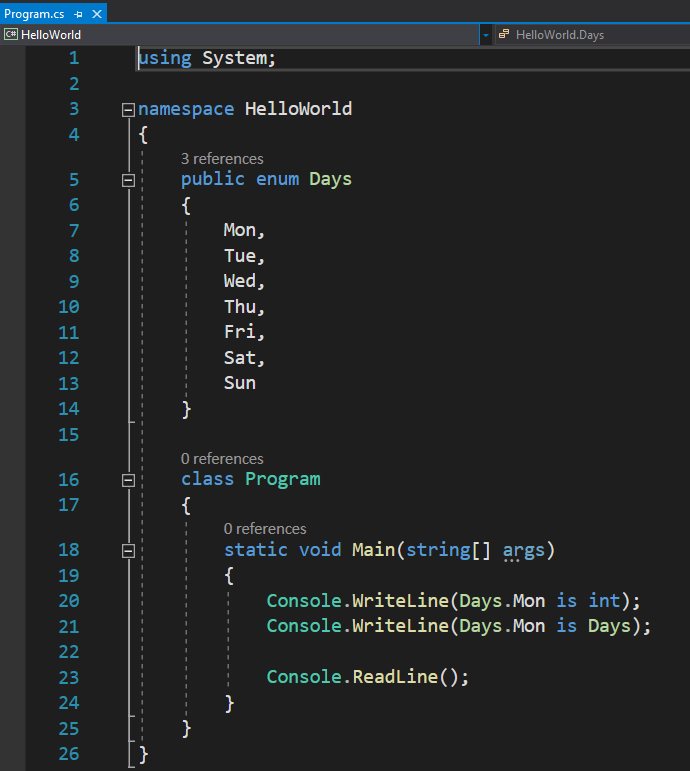
The C# keyword is is useful for checking the type of a member, returning a boolean value: true if the member is of the type we checked, or false if it’s not. So, our test will display:
because Days.Mon is not of type integer, but it is of type Days.
You may wonder why I had to cast Days.Mon to integer, and not display its value like this:
[raw][/raw]
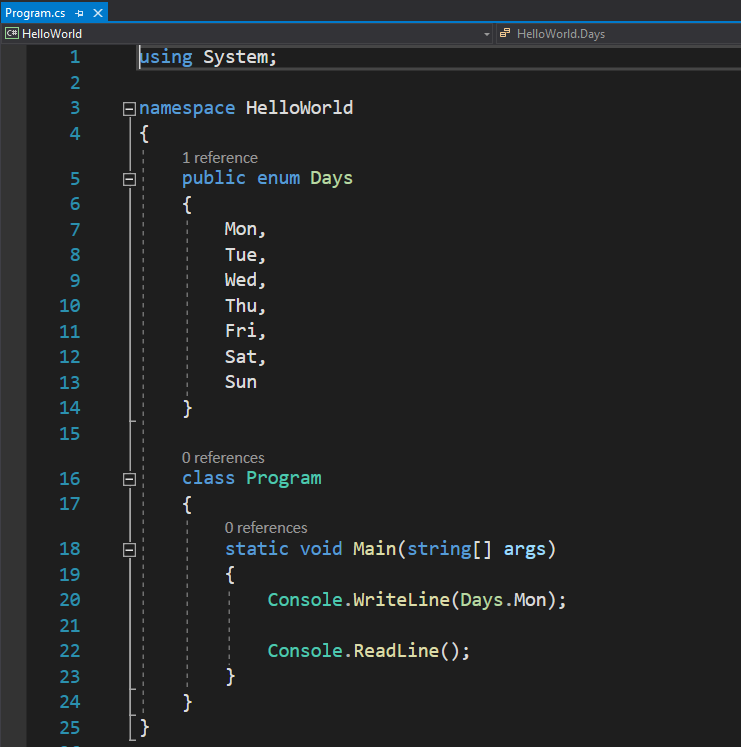
Well, if you run the above example, you will notice the output will not be 0, but Mon!
So, now I can give you the academic definition of enumerations: an enumeration is actually a textual representation of an integer. By default, this number is the constant’s index in the list of constants of a particular enumeration type.
One good thing about enumerations is that we can assign custom values to its constants:
[raw][/raw]
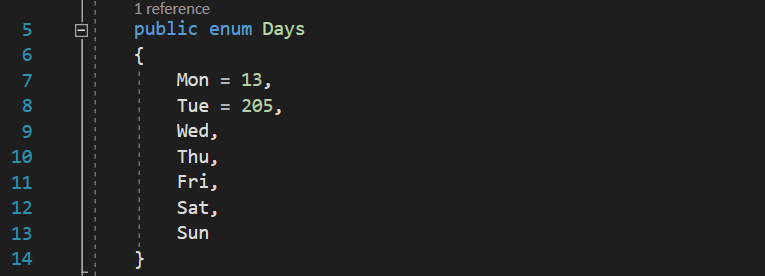
So, we learned what enumerations are, what we can do with them, etc, but how are they actually useful to us? Well, let’s consider a real life example. We have a coffee machine:
[raw][/raw]
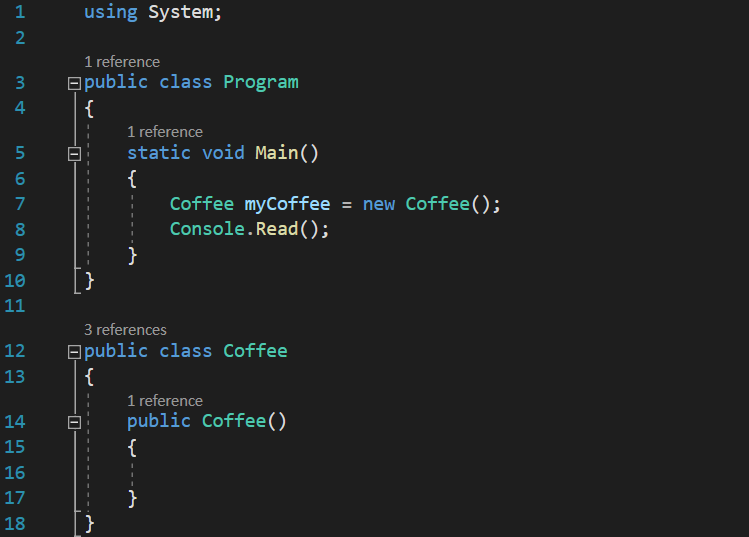
Wonderful! We just ordered a coffee! But what if we want to specify the size of our coffee? Of course, we can use a property, and set it in the constructor:
See code changes
Legend:
[/raw]
Above code works perfectly, with only one problem: the users can set whatever value they want, say 1056 ml. Sure, we can do some if-else checks in the property, sure, we can throw some errors, but that is not a very elegant solution, specially if there are a lot of cases. We could also make our quantity variable a constant, but that would also not do us any good, since we would be unable to specify the quantity we want.
And here is where enumerations come to save the day. Lets create an enumeration of the available quantities:
[raw][/raw]
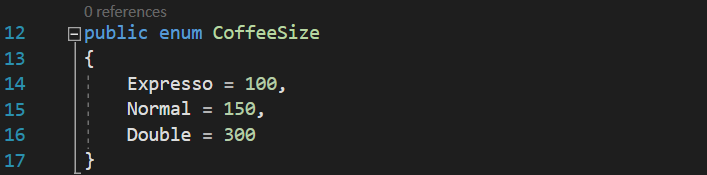
Starting to see the beauty of it? Since we said that enumerations only hold constants, the values we set to them will be the only values available to the users, and they will not be able to change them. This is exactly what we needed. So, how do we use this enumeration in our example? At the beginning of this lesson, I said that enumerations resemble classes, with the difference that they can only contain constant fields. This also means that we use enumerations the same way we use classes: using instantiation or fields/properties.
[raw][/raw]
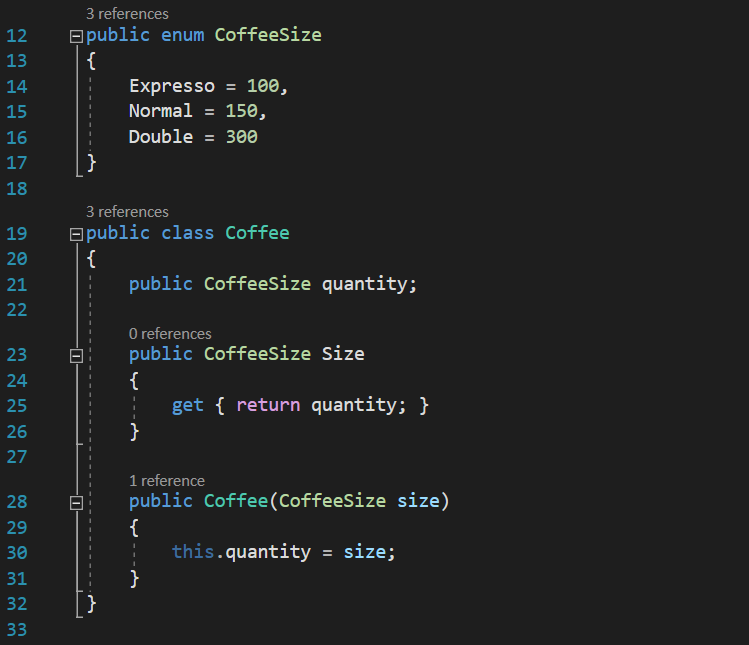
The code is rather easy to understand: we declared an enumeration called CoffeeSize, then inside our Coffee class, we declare a field and a property, both of type CoffeeSize. Finally, we set the field in the constructor of the class too. So, now we can have this kind of coffee ordering:
See code changes
Legend:
[/raw]
This is exactly what we wanted in the first place, being able to chose from a predefined set of constant values. And, yes, we cannot change the values of enum members, after declaring them:
[/raw]
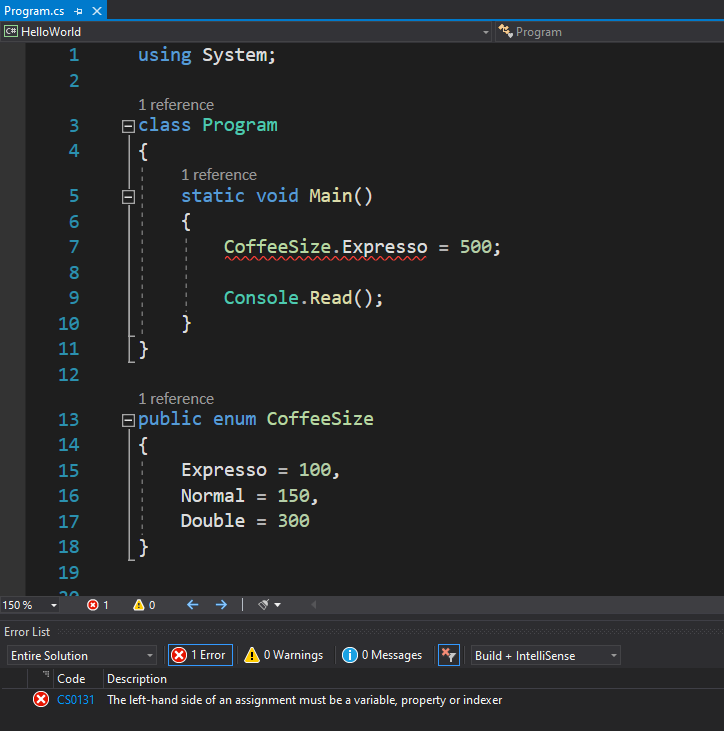
because we would get an error: The left-hand side of an assignment must be a variable, property or indexer.
One last thing that you should know about enumerations is that they are reference types, but the values inside them are value types.
EXERCISES
1. Create an enumeration BatteryType, which contains the values for the type of the battery (Li-Ion, NiMH, NiCd, …) and use it as a new field for the class Battery.
Solution
Guidelines: Use enum for the type of battery. Search in Internet for other types of batteries.
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
BatteryType myBattery = new BatteryType();
myBattery = BatteryType.NiCd;
Console.Read();
}
}
public enum BatteryType
{
LiIon,
NiMH,
NiCd
}
}
Tags: class, constants, enum, enumerations, object oriented programming, OOP