In OOP, namespaces are containers for a group of classes which have a common context or are categorized by common functionality. Namespaces do not have any kind of functionality, and it is not mandatory to use them. However, they offer a few advantages, most notably being the ability of sorting and grouping your code into logical units.… Read more
Archive for the ‘Objects’ Category
Namespaces
Wednesday, August 2nd, 2017Bit masks and the Flags enumerations attribute
Sunday, July 16th, 2017Most programmers use enums just so they can enforce a predefined set of options from which users can chose. However, enums have one major disadvantage: they can only hold one value at a time. Let’s say we have the following code:
[raw][/raw]
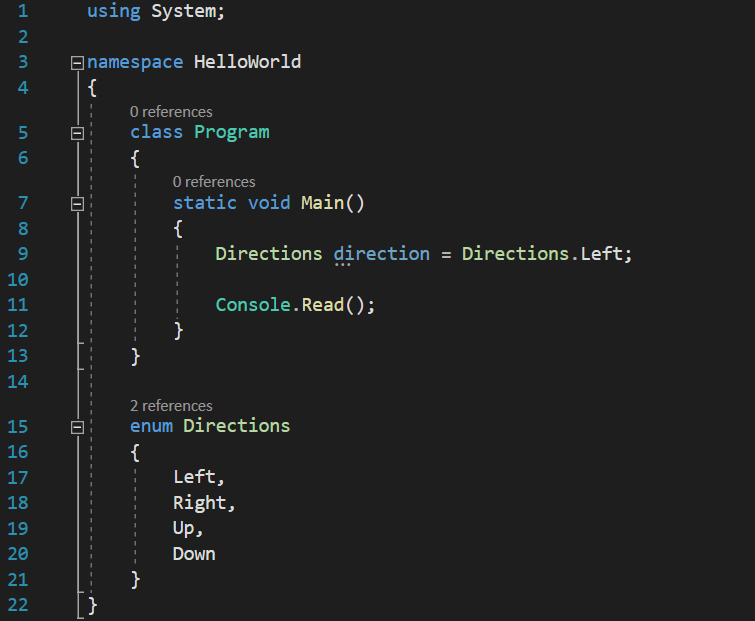
In this case, specifying that we want to have a direction towards left seems OK.… Read more
Enumerations
Sunday, July 16th, 2017Constants
Saturday, July 1st, 2017Just like constants in mathematics, C# defines special fields of classes called constants. Like their name hints, once declared and initialized, constants maintain their values, forbidding their further modification.
There are two types of constants:
- constants for which the value is set during the compilation (compile time constants)
- constants which have their value set during the execution (run-time constants)
Compile time constants are declared using the C# modifier const:
[<access_modifiers>] const <type> <name>;
A secret not many C# programmers know is that compile time constants are static fields, even if they do not contain the static keyword, and the compiler forbids its usage in the declaration.… Read more
Structures
Saturday, June 24th, 2017In C# and .NET framework, there are two implementations of the concept of “class”, from the OOP point of view: classes and structures.
While we already know that classes are defined using the class keyword, structures are defined using the keyword struct.… Read more
Generic methods
Friday, June 23rd, 2017Generic methods, like generic classes, are parameterized (typified) methods, which we use when we cannot specify the type of the method’s parameters. Also like in the case of generic classes, the replacement of unknown types with specific types happens when the method is called.… Read more
Generic classes
Sunday, June 18th, 2017Generic classes, also known as generic data types or simply generics, are classes of unknown type until they are instantiated to some specific type.
Because this concept is a bit harder to explain, I will first exemplify a specific case that will help you better understand it.… Read more
Nested classes
Friday, June 9th, 2017C# offers nested classes, which just like all the other nested programming concepts, implies a construct (class) defined inside the body of another construct (class). The class defined this way is called “inner class”, while the one which contains it, is called “outer class”.… Read more
Static members
Thursday, May 18th, 2017As we saw in the previous recent lessons, the usual way of communicating with a class is to create instances (copies) of it, and then use the resulting objects. In fact, that is the strong advantage of the classes – the ability to create copies that can be used and can be modified individually.… Read more
Access modifiers
Sunday, May 14th, 2017As their name suggests, access modifiers are some programming concepts that can alter the access level of something. In more complex words, access modifiers are reserved keywords which add information for the compiler, and the piece of code related to those modifiers.… Read more