In the previous post, I have created our first C# program, a console application that displayed a single sentence and which contained the following instructions:
[raw][/raw]
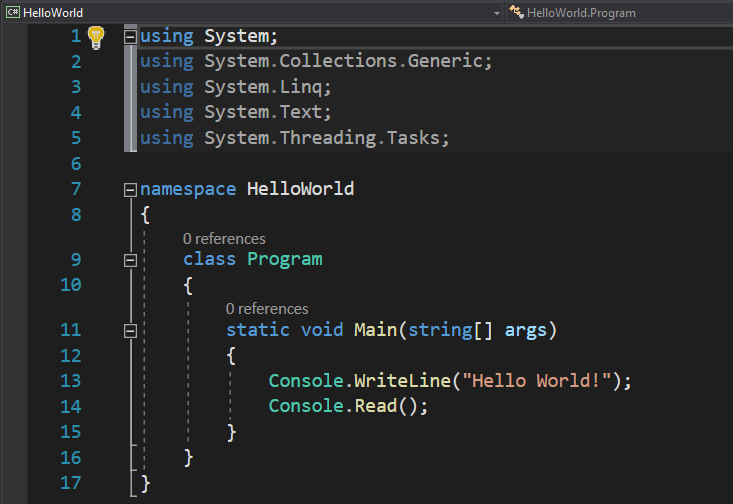
It is now the time to analyze the structure of a C# Console program.
First of all, when I say “console program”, I am not referring to game consoles. Console programs are just those black window programs that appear on the screen, they don’t contain any buttons or text boxes or images, and you can only interact with them via the keyboard.
The instructions you see in our codes are very similar to the ones you will encounter in most of the programs you will ever write.
As you can see, our program starts with a few using instructions. The using instructions ask the compiler to use the contents of a file. In the case of our program, the using instruction asks the compiler to include the contents of some files, contents which are named System.Collections.Generic, System.Text, etc.
These contents are stored in what .NET programmers refer to as namespaces. You don’t have to worry about what a namespace is for the moment, all you need to know is that they contain additional codes that we can use in our programs. Whenever you use a using directive, you don’t have to manually type those codes, and you also don’t have to re-invent the wheel.
For instance, if you would have to write a program that calculates square roots, you don’t have to create a function for doing that. Instead, you would insert using System.Math; which refers to a file that contains a function for calculating square roots, and other math functions.
As you may have noticed, a few using directives are added automatically by Visual Studio, when we created the project. Visual Studio tries to simplify your work by adding the most commonly used using directives, to get you started. However, you don’t always need all of the imported files, and this is also the reason why some of the using directives are white, while others are grayed out. Whenever we use in our programs codes that are contained in the files imported with the using directives, the used directives will turn their font color white. Therefor, whenever you see grayed out using directives, you can safely delete them.
You can even automate this process by right clicking anywhere inside the main window and choosing the option Remove and Sort Usings, like this:
Visual Studio will automatically remove the unused using directives from your program’s file.
After the using statements, you see two new concepts: namespace and class. For now, think of a class as being an object. A real world object, like a car, a house, a cat, etc. Classes are objects, and they contain properties and other stuff. Namespaces, on the other hand, are a collection of classes, grouped under the same criteria. For instance, we could have a namespace called Universe, which would contain some objects (classes): stars, planets, galaxies, etc. Now it makes sense to group those objects in a namespace, because they have something in common: they are PART of the Universe.
Next line in our program is
[raw][/raw]

Every console application that you will create will contain a line similar to the void Main instruction. In the future, you will notice that it will be far easier to group the instructions that a program has to execute so that it will be easier for you and other programmers to understand what the program does. What you have to remember is that in a Console application, the void Main function is executed first. It is the application’s entry point. So, when you double click on an executable file, the Main function is the one to be executed first.
What about the { } characters? For now, all you have to know is that C# computer programs have their instructions grouped in blocks of code, and each block starts with { and ends with }. This is how the compiler can understand where a block begins and ends. Remember that for any open curly bracket, you must use a closing curly bracket.
Finally, in our program, inside the code block of our Main method, we have two lines of code:
[raw][/raw]

By using Console.WriteLine we instructed our program to display a text in its window. By using Console.Read, we instructed it not to close the window after the execution ends (console programs tend to self close when they end their execution), but to wait for our input (in this case, keyboard text, followed by Enter key).
The concepts explained in this lesson are also shown visually in the following video: